Breadth First Search
Breadth First Search is used to compute the distance (in terms of the number of edges) from a given source node in a graph to all other nodes in the graph and can be used on both directed and undirected graghs. The general idea is to start at a source node and then look at all of its neighbors. After you have looked at the source's neighbors, you look at the neighbors of the sources neighbors, and so on. With each iteration, the frontier of "discovered" nodes expands radially outward from the original source.
To implement a Breadth First Search, a few additional data structures are needed:
- an array to hold parent information ( pi[#vertices] )
- an array to hold color information ( color[#vertices] )
- an array to hold distance information ( d[#vertices] )
- a queue ( Q )
The pseudo-code for BFS:
- for each vertex in the graph except for the source vertex:
- set its color to white
- set its parent to NIL
- set its distance to infinity
- for the source vertex:
- set its color to grey
- set its parent to NIL
- set its distance to 0
- put the source vertex into the queue
- while the queue isn't empty...
- take an element (this is a vertex, lets call it u) out of the
queue
- for every neighbor of the vertex u (lets call that neighbor
v)
- if the color of the neighbor ( v ) is white...
- set its color to grey
- set its parent equal to the vertex u
- set its distance equal to the distance of its parent
plus 1
- put the vertex ( v ) into the queue
- if the color of the neighbor ( v ) isn't white, do
nothing
- remove u from the queue
- change u's color to black
The following is an example of BFS on a directed graph with ( c ) as the source vertex. The letter next to each vertex represent the name of that vertex, the text inside the vertex correspond to the vertex's distance information. The parent information is not included.
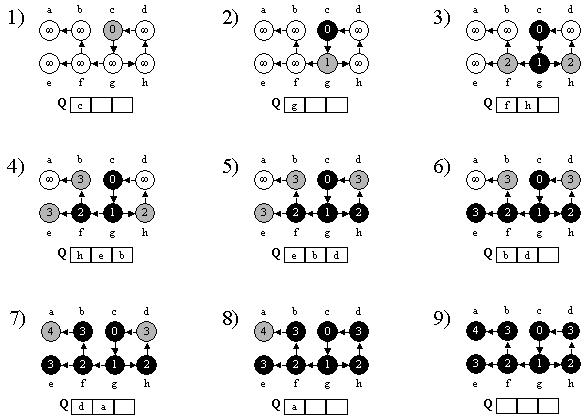
Tim Mauch
Last modified: Fri Sep 26 16:51:00 PDT 2003