
|
|
Top

|
- Machine languages - Natural language of a computer, sequence of 1's and 0's. No translation
necessary, ready for immediate execution. (different for each type of computer (machine-dependent)).
- Assembly languages - English-like abbreviations for elementary operations (assembler converts
them to machine language).
- High-Level languages - English-like statements that perform many operations in a single
statement (compilers translate them into machine language).
A program written in a high-level language is called a source program.
A program with machine language instructions is called an object program.
A compiler translates high-level code into machine language.
|
Top

|
- Edit - write program
- Preprocess - process code (include other files)
- Compile - create object code (machine language code)
- Link - links object code with libraries and creates an executable
- Load - load into main memory
- Execute - run the program
|
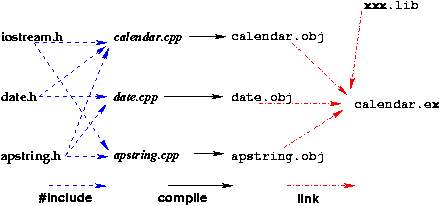
Figure 1: Steps 2,3,4 of running a program.
|
Top

|
- Syntax Errors (compile-time) - - Program does not complete compilation.
Violations of syntax rules which define how the language must be written.
Compilation error messages
1 void main()
2 {
3 double number;
4 cout << "Enter number: ";
5 cin >> number;
6 inverse = 1 / number
7 }
undefined symbol 'cout' - line 4
undefined symbol 'inverse' - line 6
parse error - line 6
- Semantic Errors (run-time) - -Program does not finish running.
Program crashses while running.
Caused by instructions that ask the computer to do something illegal.
Analyze error messages and trace through the program.
Ex. divide by zero
- Logic Errors - Program compiles and runs, but does not perform the expected task correctly.
Errors when designing the program specifications or what you asked the computer to do was different than what you intended to ask the computer to do.
Review the problem over again.
Ex. wrote x < y meant x <= y
|
Top

|
(See “C++ Style Guidelines” handout posted on Pr.Kelsey web page.)
- Use blank lines to separate set of related instructions.
- Liberally use clear and helpful comments.
- Make comments stand out - end of line (lined up) or blank line above comment.
- Type each statement on a separate line.
- Avoid running a statement over multiple lines.
- Avoid line splicing (line too long)
- Indent lines after a curly brace by the same amount until the end curly brace.
- Use whitespace in typing statements (cout<<"Hello all"; cout << “Hello all”;)
|
Top

|